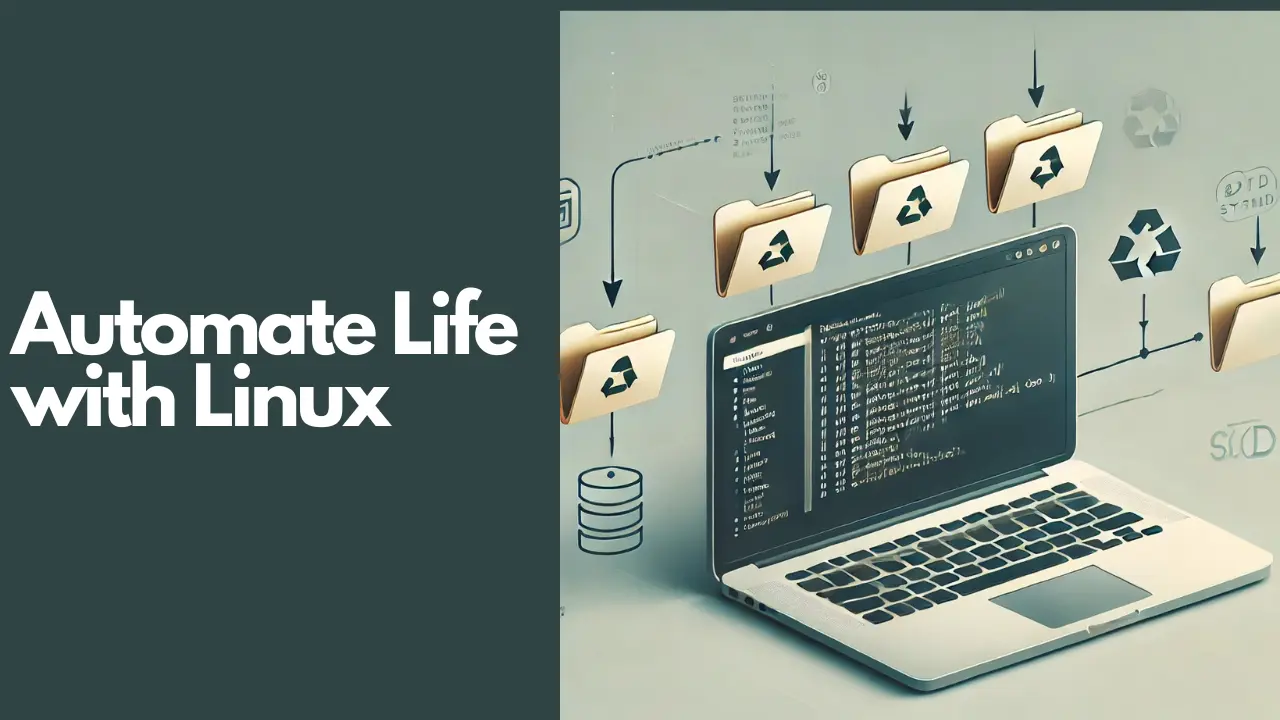
Automate Life with Linux
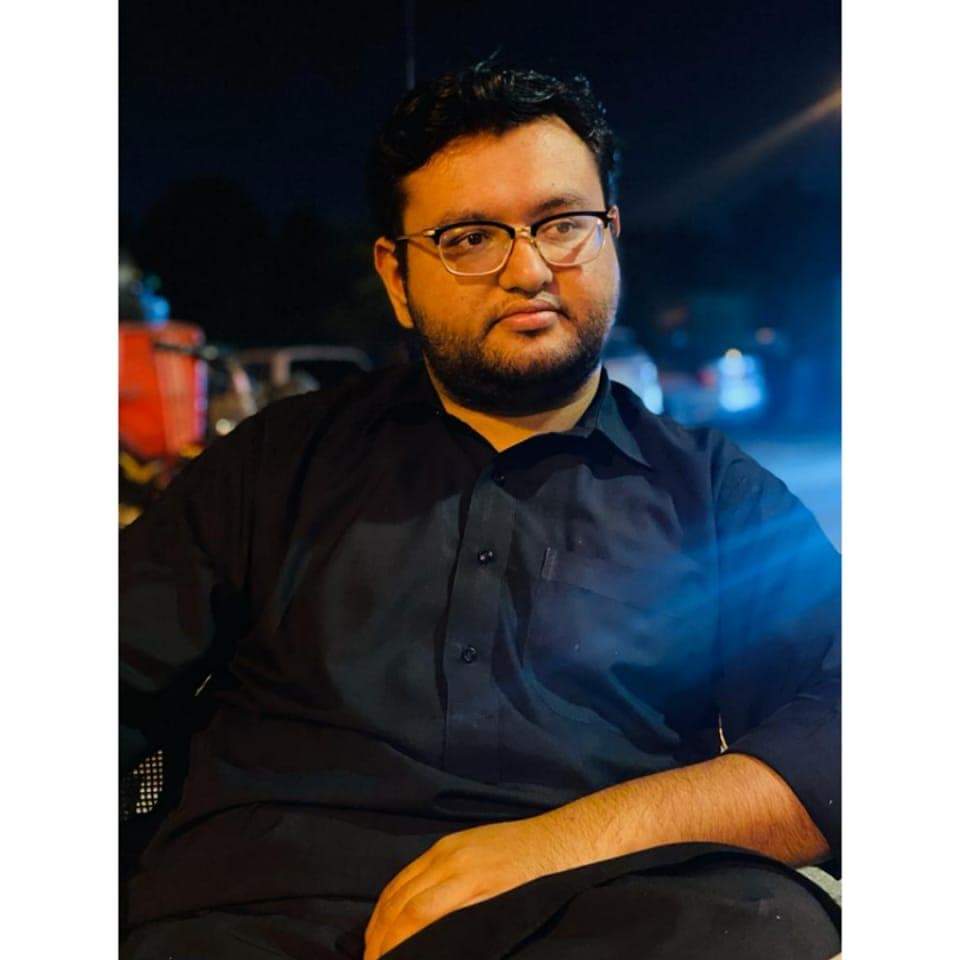
AI Article Summary
I’ve been using Linux for the past five years, and during this time, I’ve learned a lot. Every distribution might operate similarly, but each has unique characteristics that make it special. Regardless of the distribution I’m using, one of my key practices is creating my own services and automating tasks.
Writing scripts and enabling them as services has streamlined my workflow and made my life easier. One of my favorite automations is file management.
File management can be tricky, so I’ve created a script to handle three main types of files: Git repositories, images, and zip files. This script automatically moves these files to designated locations—specifically, the Documents
and Pictures
folders.
Now, let’s break down the script line-by-line, then set it up to run on our system as a systemctl
service.
Writing the Code
Let’s go over the key sections of our Bash script.
Setting Destination Folders
First, we define the destination folder paths for Git repositories and images. These paths will be used throughout the script to move files.
# Set the destination folder paths
gitDestination="$HOME/Documents/Testing-Repo"
imageDestination="$HOME/Pictures/Images"
Functions for Moving Images and Git Repositories
Next, we create two functions to handle moving image directories and Git repositories. The moveImages()
function moves images from a specified folder to the image destination folder, while the moveGitRepo()
function moves Git repositories to the Git destination. These functions perform necessary checks before moving the files.
# Function to move images to the image destination folder
moveImages() {
# Code snippet for moving images
}
# Function to move Git repositories to the Git destination folder
moveGitRepo() {
# Code snippet for moving Git repositories
}
Checking and Moving Existing Files
The script begins by checking if the destination folders for Git repositories and images exist. If not, it creates them using mkdir -p
. Then, it moves existing Git repositories (excluding those containing images) and image files from the Downloads folder to the appropriate destination folders.
# Check if the Git destination folder exists; if not, create it
if [ ! -d "$gitDestination" ]; then
mkdir -p "$gitDestination"
echo "✅ Created destination folder for Git repositories: $gitDestination"
fi
# Move existing Git repositories to the destination folder, excluding those with images
find "$HOME/Downloads" -maxdepth 1 -type d -name "*.git" -exec sh -c '
repoDir=$(dirname "$0")
if ! find "$repoDir" -type f -exec file -N -i -- {} + | grep -qE "image/"; then
moveGitRepo "$repoDir" "$gitDestination"
fi
' {} \;
Checking if the destination folder exists for images and creating it if it doesn’t:
if [ ! -d "$imageDestination" ]; then
mkdir -p "$imageDestination"
echo "✅ Created destination folder for images: $imageDestination"
fi
moveImages "$HOME/Downloads" "$imageDestination"
Live Monitoring for New Files
The script then enters a live monitoring loop using inotifywait
. It waits for new directories to be created in the Downloads folder and performs actions based on the type of the new directory. If it’s a Git repository without images, it waits for 3 minutes and then moves it to the Git destination folder. If it’s an image directory, it moves it to the image destination folder.
# Start live monitoring of the Downloads folder for new directory creations using inotifywait
inotifywait -m -e create -r --format "%w%f" -q "$HOME/Downloads" |
while read -r directory; do
# Check if the newly created directory is a Git repository
if [ -d "$directory/.git" ]; then
# Check if the Git repository contains images
if ! find "$directory" -type f -exec file -N -i -- {} + | grep -qE "image/"; then
# Move the Git repository to the Git destination folder after 3 minutes
moveGitRepo "$directory" "$gitDestination" &
fi
elif file -N -i "$directory" | grep -qE 'image/'; then
# Check if the newly created directory is an image
# Move the image directory to the image destination folder
moveImages "$directory" "$imageDestination"
fi
done
Running the Script as a System Process
To run the script as a background process whenever the system starts, we’ll create a systemd
service. This enables the script to continuously monitor files and perform actions.
- Create a service file for the script:
sudo nano /etc/systemd/system/file-management.service
- Add the following content to the file:
[Unit]
Description=File Management Script
After=network.target
[Service]
ExecStart=/bin/bash /path/to/your/script.sh
Restart=always
[Install]
WantedBy=multi-user.target
Replace /path/to/your/script.sh
with the actual path to your script.
- Save the file and exit.
- Reload
systemd
to read the new service:
sudo systemctl daemon-reload
- Enable the service to start on boot:
sudo systemctl enable file-management.service
- Start the service:
sudo systemctl start file-management.service
- Verify that the service is running:
sudo systemctl status file-management.service
Your script is now running as a system process. It will start automatically on system boot and monitor files in the background.
Conclusion
With this introduction to systemd
and systemctl
services, you can see how easy it is to create services that automate routine tasks. This is just the beginning—you can create services to manage videos, images, Git repositories, system snapshots, and much more. So be creative, write some cool scripts, and share your work with others!
Until next time, keep coding, nerds!
💬 Join the Discussion
Share your thoughts and engage with the community